In this tutorial let us create a simple application to demonstrate the use of entity framework using code first.
We are using Visual Studio 2015 and entity framework 6.1.3. You can download Visual Studio community Edition . You should have the basic knowledge of .Net framework, C# and MS SQL Server.
In this tutorial, we will create a simple application with a user class. Our user class will have basic information like name and email address of the user.
Create the Project
- Open Visual Studio.
- File ->New -> Project
- Select C# ->
- Select Console Application
- Name the application as “EFGettingStarted”
- Click on OK
Install Entity Framework
The next step is to install the Entity framework. This can be installed via nuget package console. Click on Tools->Nuget Package manager -> Package Manager Console and type the following command
This will install the latest version of the Entity Framework (6.1.3).
Entity Type
The next step is to create the Entity Data Model (EDM)
- Select the Project
- Right click and click on add -> Class
- Name the class as Model.cs
- Copy the following code to the model.cs
We are done with our entity model.
DBContext
The DbContext (often referred as context) is the class which is responsible for interacting with the entity model and the data store. It allows you to query, insert, update and delete operations on the entities. This class is derived from the system.data.entity.dbcontext namespace. The older version of the entity framework used objectContext. DbContext is actually a wrapper around the objectContext class.
DbContext is responsible for the following
Database
DbContext is responsible for creating the Database instance for the context. It allows you to create,delete, check for the existence of the underlying database.
Connections
DBcontext manages connections to the database. It Opens the connections when needed and closes it when finished the processing of the query
Entity Set
DbContext exposes the property (DbSet<TEntity>) which represent collections of entities in the context. DbContext manages these entities during the lifetime of the entities.
Querying
DbContext converts LINQ-to-Entities queries to SQL query and sends it to the database.
Change Tracking
DbContext tracks the changes done to the each entity in its lifetime. It maintains the state of each entity (Added, Unchanged, Modified, deleted and detached).
Persisting Data
DbContext performs the CRUD operations to the database to persist the data.
Caching
DbContext does first level caching by default. It stores the entities which have been retrieved during the lifetime of a context class.
Manage Relationship
DbContext also manages associations between using data annotations/fluent API.
Materialization
DbContext translates the results returned by the underlying database into entities. This process called as materialization
Configuration
DBContext Provides API to which allows us the configure the behavior of the context.
Creating the Context
You can add a DbContext in your project by creating a class which derives from the DbContext class
- Select the Project
- Right Click -> Add -> Class
- Name the class as EFContext.cs
- Click on Add
- Import the namespace System.Data.Entity
- Make the EFContext class as public and inherit it from the DbContext class
Now we have added the Context class EFContext to our project
DBSet
As mentioned above DbContext class exposes the generic version of DbSetwhich represents the collection of entities (entity set). Each entity type must expose the DbSet property so that they can be used in CRUD Operations
DbSet provides the methods to manage the entity set. To Add DbSet Property to your class add the following in the context class
Finally, our EFContext class looks like this
Adding data
We have defined our model (user class) and the context (EFContext). Now it is time to create a new user
Select the program class
In the main method of the class, paste the following code
In the main method of the class, paste the following code
In the above code, we created a new user instance. Then, we created an instance of DbContext object. The DbContext returns the Users Entity set (DbSet<TEntity>). We invoked the add method of the Users object and passed the newly created user instance. Finally, we invoked SaveChanges method of the DbContext object to save the changes to the database.
Now we are ready to test our application. Run the Application. If everything is ok then you should see “press any key to close” message. Press any key and console window will close.
Where is the database
We have not defined our database connection string. The Code first smart enough to create the database. Code First uses a series of steps to find and initialize the database. The Process is called database initialization.
Code First creates the database in localdb. (In older version of Visual studio SQL Server Express).
In Visual Studio go to View->SQL Server Object Explorer. You should see (localdb)\V11.0 under the SQL Server. (If you have installed SQL Server 2014 or Visual Studio 2015, then the localDb is available at (localdb)\MSSQLLocalDB). Expand the database and You will see that the database by the name “EFGettingStarted.EFContext” is created.
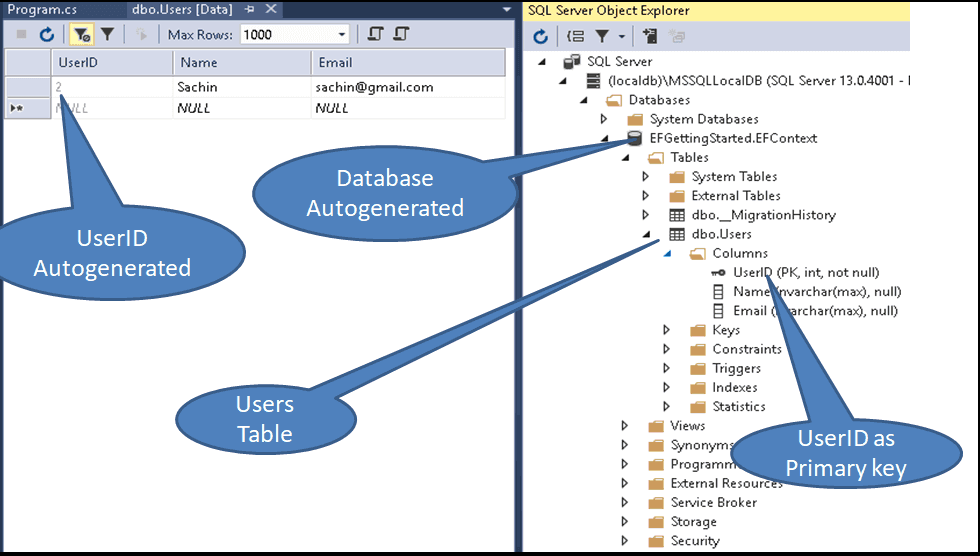
As you can see from the above image, Entity Framework has created users table with the column for each property. Few important things to note that
- Database was automatically created by EF
- Name of the database is “EFGettingStarted.EFContext”, which is fully qualified name of DbContext object
- Table names are pluralized. Our Entity class name was User. Table name is Users
- Column UserID is automatically configured as Primary Key.
- The UserID is also configured as identity column
The Code First does this by using Code-first conventions. It uses the model to create the database and build the tables and columns of the tables.
Conclusion
In this tutorial, we have created a simple application and learned how entity framework works
R b88.bet - The Top Bet on Sports
ReplyDeleteR b88.bet is 바카라사이트 a sports betting website that 우리카지노 was established in 2003. In the past year, it has also received a number of significant ratings and reputation rb88 awards