Consider the class
Invoke the above from your form using
This will show “Hello” in message box.
Consider the scenario where you don’t have the access to the above code and you want to add another method to the above class. That is where the C# feature of extension method is to be used.
Consider the scenario where you don’t have the access to the above code and you want to add another method to the above class. That is where the C# feature of extension method is to be used.
Extension methods
Extension methods as the name implies, is about extending the functionalities of the class. The Extension methods help you add a new method to the existing Class (or Type).
Now let us add the new method sayHI to the above testclass . First create a new static class and name it as testExtension. Remember that the extension method must be defined as static method
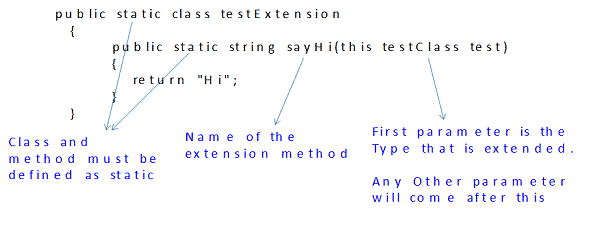
Now you can use sayHI method just like any other method as shown below.
1
2
3
4
5
6
7
|
testClass test = new testClass();
MessageBox.Show( test.sayHello());
MessageBox.Show(test.sayHi());
|
Few Important things to note
- Extension method must be defined as static method and must reside in a static class
- this keyword must be the first parameter of the extended method. This will actually inform the compiler that the method is an extension method.
- Extension method must be defined in the root of the namespace. It cannot be nested inside another class under the namespace
- The type that is being extended must be referenced in the extension method. Use the using statement to import the namespace.
- protected/private members of the type that is being extended will not be accessible from the Extension methods.
- You cannot override any method of the type you are extending.
- The Extension method has lower priority than the methods defined In the type. Similar named method in instance, always executed rather than the extended method. In fact the extended method will never be called
- The Extension methods with the same name and signature may signature for the same class may be declared in multiple namespaces without causing compilation errors.
- Extension method cannot be used for adding a new properties, fields or events
Benefits
- Extension methods appear under the intellisense.
- It makes the code more readable.
- Extend the functionality of third party libraries where you don’t have access to the code. ( This may also break your code if the third party vendor changes the implementation of the library)
Conclusion
Extension methods are nice little feature of c#. LINQ is one module which uses extension methods extensively.
Thanks for this blog its useful
ReplyDeleteThanks for the blog. Looking forward for more info on C# and more technologies...
ReplyDeleteThanks for this blog its useful
ReplyDeleteReally nice blog and it's very use full for beginners as well as exp. thanks mate for such a nice blog..keep it up...
ReplyDeleteReally nice blog and it's very use full for beginners as well as exp. thanks mate for such a nice blog..keep it up...
ReplyDelete